简介
XStream是Java类库,提供了所有的基础类型、数组、集合等类型直接转换的支持,用来将对象序列化成XML或反序列化为对象。
基本使用
XStream反序列化分析采用依赖版本如下:
1 2 3 4 5
| <dependency> <groupId>com.thoughtworks.xstream</groupId> <artifactId>xstream</artifactId> <version>1.4.10</version> </dependency>
|
定义接口类IndexInterface如下:
1 2 3 4 5 6
| package org.example.deserialize.xstream;
public interface IndexInterface {
void output(); }
|
定义类Index实现IndexInterface接口如下:
1 2 3 4 5 6 7 8 9 10
| package org.example.deserialize.xstream;
public class Index implements IndexInterface {
String name; @Override public void output() { System.out.println("Hello, " + this.name); } }
|
定义类IndexXML实现XStream序列化与反序列化如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| package org.example.deserialize.xstream;
import com.thoughtworks.xstream.XStream; import com.thoughtworks.xstream.io.xml.DomDriver; import java.io.FileInputStream;
public class IndexXML {
public static void main(String[] args) throws Exception { serialize(); deserialize(); }
public static void serialize() { Index index = new Index(); index.name = "XStream"; XStream xStream = new XStream(new DomDriver()); String xml = xStream.toXML(index); System.out.println(xml); }
public static void deserialize() throws Exception { FileInputStream fileInputStream = new FileInputStream("/Users/alphag0/Desktop/Code/Java/JavaSecCode/src/main/java/org/example/deserialize/xstream/index.xml"); XStream xStream = new XStream(new DomDriver()); Index index = (Index) xStream.fromXML(fileInputStream); index.output(); } }
|
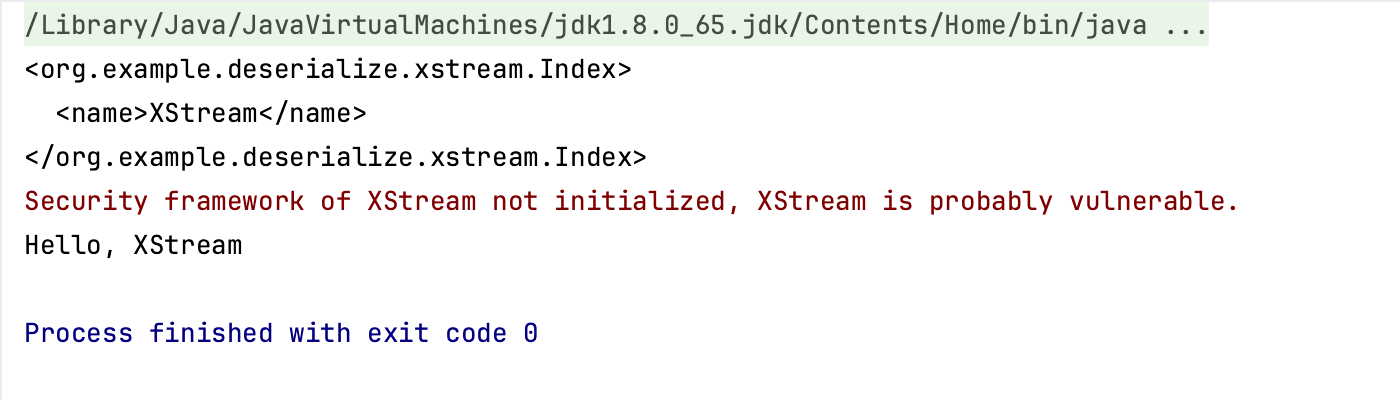
前置知识
Converter
Converter的职责是提供一种策略,用于在对象图中找到的特定类型的对象与XML之间的转换,XStream为Java的常见类型(原始类型、字符串、文件、集合、数组和日期等)提供了Converter转换器。简而言之,就是输入XML后它能识别其中的标签字段并转换为相应的对象,反之亦然。
转换器需要实现的三个方法:
- canConvert方法:告诉XStream对象,它能够转换的对象
- marshal方法:将对象转换为XML时候的具体操作
- unmarshal方法:将XML转换为对象时的具体操作
MapConverter
MapConverter是针对Map类型还原的Converter,跟进com.thoughtworks.xstream.converters.collections#unmarshal方法,依次调用unmarshal方法、populateMap方法和putCurrentEntryIntoMap方法,在putCurrentEntryIntoMap方法中会调用Map#put方法,后续就是对key调用hashCode函数。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| protected void populateMap(HierarchicalStreamReader reader, UnmarshallingContext context, Map map) { this.populateMap(reader, context, map, map); }
protected void populateMap(HierarchicalStreamReader reader, UnmarshallingContext context, Map map, Map target) { while(reader.hasMoreChildren()) { reader.moveDown(); this.putCurrentEntryIntoMap(reader, context, map, target); reader.moveUp(); }
}
protected void putCurrentEntryIntoMap(HierarchicalStreamReader reader, UnmarshallingContext context, Map map, Map target) { reader.moveDown(); Object key = this.readItem(reader, context, map); reader.moveUp(); reader.moveDown(); Object value = this.readItem(reader, context, map); reader.moveUp(); target.put(key, value); }
|
TreeSetConverter&TreeMapConverter
TreeSetConverter的反序列化处理方式为先转化为TreeMapConverter的方式,优先还原TreeSet里的TreeMap,再填充到TreeSet中。
从TreeSetConverter的调用来看看整个的调用过程,先从TreeSet中提取出TreeMap,接着调用TreeMapConverter来还原TreeMap。在TreeMapConverter中利用sortedMap来填充需要还原的Entry,这里会回到上文提到的MapConverter类中的populateMap和putCurrentEntryIntoMap方法,最后调用TreeMap#putAll方法,调用到java.util.AbstractMap#putAll方法。